There is a educational need for Ukrainian students to utilize the Ukrainian Phonetic keyboard when using their chrome book to write documents or papers. That being said, there was no Ukrainian phonetic chrome extension available and the one that was provided here: https://github.com/nagornyi/ukrainian-phonetic-keyboard-chromeos did not fulfill the requirements needed for students using a particular chrome book. As such, we needed to create our own chrome extension.
Here is the code I used:
//Manifest
{
"name": "Ukrainian Phonetic Keyboard",
"short_name": "UKrainian Phonetic Keyboard",
"version": "1.3",
"manifest_version": 3,
"description": "Ukrainian Phonetic keyboard layout for Chrome OS with custom key mappings. Custom mappings and auto context refresh.",
"permissions": [
"input"
],
"background": {
"service_worker": "background.js"
},
"input_components": [
{
"name": "Ukrainian Phonetic",
"type": "ime",
"id": "ukrainian-phonetic",
"description": "Ukrainian Phonetic keyboard layout",
"language": "uk",
"layouts": ["ua(phonetic)"]
}
]
}
//Background Script
let currentContextID = null;
chrome.input.ime.onFocus.addListener((context) => {
currentContextID = context.contextID;
console.log('Focus event handled. currentContextID set to:', currentContextID);
});
chrome.input.ime.onKeyEvent.addListener((engineID, keyData) => {
const { code, type } = keyData;
if (type !== 'keydown' || !currentContextID) {
return false;
}
const keyMap = {
'KeyA': 'а', 'KeyB': 'б', 'KeyC': 'ц', 'KeyD': 'д',
'KeyE': 'е', 'KeyF': 'ф', 'KeyG': 'ґ', 'KeyH': 'г',
'KeyI': 'і', 'KeyJ': 'й', 'KeyK': 'к', 'KeyL': 'л',
'KeyM': 'м', 'KeyN': 'н', 'KeyO': 'о', 'KeyP': 'п',
'KeyQ': 'я', 'KeyR': 'р', 'KeyS': 'с', 'KeyT': 'т',
'KeyU': 'у', 'KeyV': 'в', 'KeyW': 'ш', 'KeyX': 'х',
'KeyY': 'и', 'KeyZ': 'з', 'Digit1': '1', 'Digit2': '2',
'Digit3': '3', 'Digit4': '4', 'Digit5': '5', 'Digit6': '6',
'Digit7': '7', 'Digit8': '8', 'Digit9': '9', 'Digit0': '0',
'Minus': '-', 'Equal': 'є', 'Comma': ',', 'Period': '.',
'Space': ' ', 'Enter': '\n', 'Semicolon': ';', 'Slash': 'ь',
'Quote': "'", 'Backquote': '`', 'BracketLeft': '[',
'BracketRight': 'ї', 'Backslash': '\\'
};
if (keyMap[code]) {
if (currentContextID) {
chrome.input.ime.commitText({
contextID: currentContextID,
text: keyMap[code]
});
return true;
} else {
return false;
}
}
return false;
});
Logic for the code above:
manifest.json: I added input permissions and a background service worker association to the background.js script file. I added metadata information such as name, shortname and description for chrome extension publishing requirement needs.
background.js: I globally set the currentContextID as this changes every time a user clicks on a different text view. Using the chrome.input.ime.onFocus listener we are able to set the global context to the most recent context. Using the chrome.input.ime.onKeyEvent listener, we are able to just condition for key down events and otherwise, we would just return default behavior. Within the keyEvent listener, we have a dictionary with all the custom mappings and a handler that will commit text to the current context. The text that is committed comes from either the custom code mapping or the default code mapping, depending on whether the pressed key is in the custom mapping dictionary.
To publish the chrome extension, I signed into my Chrome Web Store Developer Dashboard after verifying my account for publishing.

I Zipped my manifest and backgroud file making sure to remove any comments and logs. I then created a new item by copying over my Zipped file to this developer dashboard. I filled out the store listing, privacy and distribution information that is required for publishing and submitted it for review.
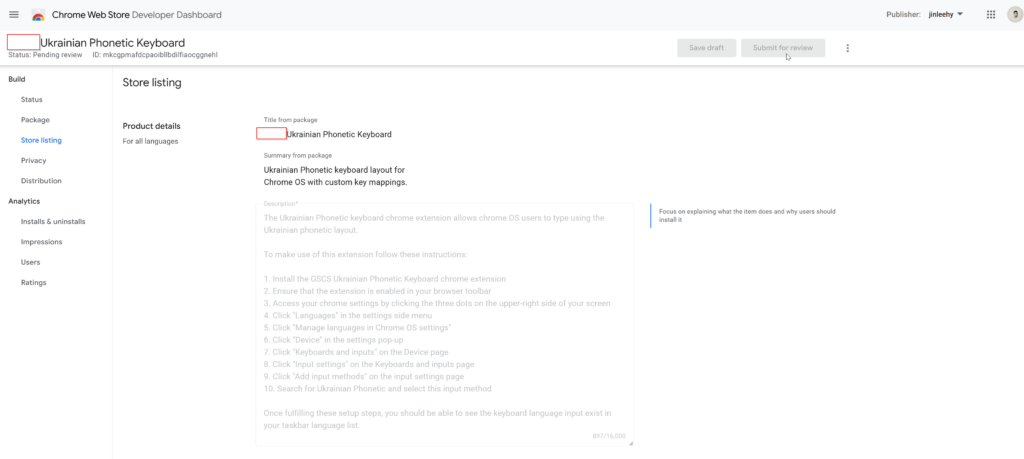
Currently, it is pending for review. These are the instructions that I have given to the client to leverage this chrome extension:
- Install the GSCS Ukrainian Phonetic Keyboard chrome extension
- Ensure that the extension is enabled in your browser toolbar
- Access your chrome settings by clicking the three dots on the upper-right side of your screen
- Click “Languages” in the settings side menu
- Click “Manage languages in Chrome OS settings”
- Click “Device” in the settings pop-up
- Click “Keyboards and inputs” on the Device page
- Click “Input settings” on the Keyboards and inputs page
- Click “Add input methods” on the input settings page
- Search for Ukrainian Phonetic and select this input method